NoomioJS
Javascript Examples §
Create a file name app.js and place it in working directory or use the Interactive Console to send the file directly (See API).
Hello World §
Let's start with outputing text to stdout with the print function.
Port Name | Baud Rate |
---|---|
COM (DEBUG) | 115200 |
Hardware Setup §
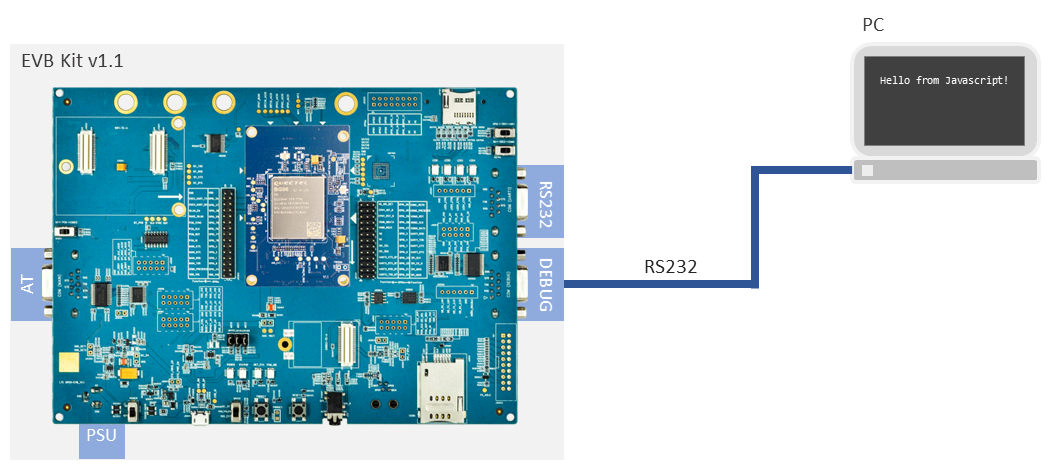
Javascript Code §
print('Hello from Javascript!');
Timer §
The example below uses the DigitalOut, Timer and print API. The function print is used to output information over the serial.
Hardware Setup §
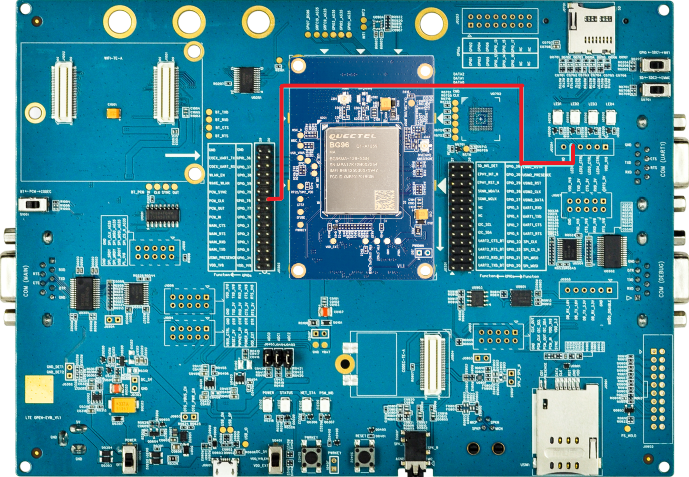
Javascript Code §
var count = 0; var intervalId; dout = DigitalOut(PIN4); var val = false; setTimeout(function cb() { print('one shot timer, called after 10 seconds'); }, 10000); intervalId = setInterval(function () { print('interval', ++count); val = !val; dout.write(val); if (count >= 25) { clearInterval(intervalId); } }, 1000); print("Hello from Javascript!");
Note that execution order is:
Hello from Javascript!
interval1
interval2
...
interval10
one shot timer, called after 10 seconds
interval11
...
interval25
I2C §
The example uses the I2C API to communicate with a MCP9808 sensor and print every 1 second the measured temperature.
var mcp9808 = { slave_address: 0x18, methods: { request_id: 0x06, request_temperature: 0x05 }, send_buffer: Uint8Array.allocPlain(1), receive_buffer: Uint8Array.allocPlain(2), frequency: 150 }; mcp9808.send_buffer[0] = mcp9808.methods.request_temperature; var tick; var interval = 1000; const sensor = i2c(I2C1); sensor.frequency(mcp9808.frequency); sensor.on('open', function () { tick = setInterval(function () { sensor.transfer(mcp9808.slave_address, mcp9808.send_buffer, mcp9808.receive_buffer); }, interval); sensor.on('data', function(data) { mcp9808.receive_buffer = data; data[0] = data[0] & 0x1F; var signed = data[0] & (1 << 5) ? -1 : 1; var temperature = signed * (data[0] * Math.pow(2, 4) + data[1] * Math.pow(2, -4)); var symbol = signed ? '+' : '-'; var message = [ 'Raw data received', 'MSB:' + data[0], 'LSB:' + data[1], 'Signed:', signed, 'Temperature:', symbol, temperature, 'C' ].join(' '); print(message); }); });